raw_storage_iterator<ForwardIterator, T>
 |
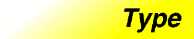 |
 |
|
 |
|
Categories: allocators, iterators, adaptors |
Component type: type |
Description
In C++, the operator new allocates memory for an object and then
creates an object at that location by calling a constructor. Occasionally,
however, it is useful to separate those two operations. [1] If i is an
iterator that points to a region of uninitialized memory, then you
can use construct to create an object in the location pointed to
by i. Raw_storage_iterator is an adaptor that makes this
procedure more convenient. If r is a raw_storage_iterator, then
it has some underlying iterator i. The expression *r = x is
equivalent to construct(&*i, x).
Example
class Int {
public:
Int(int x) : val(x) {}
int get() { return val; }
private:
int val;
};
int main()
{
int A1[] = {1, 2, 3, 4, 5, 6, 7};
const int N = sizeof(A1) / sizeof(int);
Int* A2 = (Int*) malloc(N * sizeof(Int));
transform(A1, A1 + N,
raw_storage_iterator<Int*, int>(A2),
negate<int>());
}
Definition
Defined in the standard header memory, and in the nonstandard
backward-compatibility header iterator.h.
Template parameters
Parameter
|
Description
|
Default
|
OutputIterator
|
The type of the raw_storage_iterator's underlying iterator.
|
|
T
|
The type that will be used as the argument to the constructor.
|
|
Model of
Output Iterator
Type requirements
-
ForwardIterator is a model of Forward Iterator
-
ForwardIterator's value type has a constructor that takes a
single argument of type T.
Public base classes
None.
Members
Member
|
Where defined
|
Description
|
raw_storage_iterator(ForwardIterator x)
|
raw_storage_iterator
|
See below.
|
raw_storage_iterator(const raw_storage_iterator&)
|
trivial iterator
|
The copy constructor
|
raw_storage_iterator& operator=(const raw_storage_iterator&)
|
trivial iterator
|
The assignment operator
|
raw_storage_iterator& operator*()
|
Output Iterator
|
Used to implement the output iterator expression *i = x. [2]
|
raw_storage_iterator& operator=(const Sequence::value_type&)
|
Output Iterator
|
Used to implement the output iterator expression *i = x. [2]
|
raw_storage_iterator& operator++()
|
Output Iterator
|
Preincrement.
|
raw_storage_iterator& operator++(int)
|
Output Iterator
|
Postincrement.
|
output_iterator_tag iterator_category(const raw_storage_iterator&)
|
iterator tags
|
Returns the iterator's category.
This is a global function, not a member.
|
New members
These members are not defined
in the Output Iterator requirements,
but are specific to raw_storage_iterator.
Function
|
Description
|
raw_storage_iterator(ForwardIterator i)
|
Creates a raw_storage_iterator whose underlying iterator is i.
|
raw_storage_iterator& operator=(const T& val)
|
Constructs an object of ForwardIterator's value type at the
location pointed to by the iterator, using val as the
constructor's argument.
|
Notes
[1]
In particular, this sort of low-level memory management is used
in the implementation of some container classes.
[2]
Note how assignment through a raw_storage_iterator is implemented.
In general, unary operator* must be defined so that it returns a
proxy object, where the proxy object defines operator= to perform
the insert operation. In this case, for the sake of simplicity, the
proxy object is the raw_storage_iterator itself. That is, *i
returns i, and *i = t is equivalent to i = t. You should not,
however, rely on this behavior. It is an implementation detail,
and it is not guaranteed to remain the same in future versions.
See also
Allocators, construct, destroy, uninitialized_copy
uninitialized_fill, uninitialized_fill_n,
Copyright ©
1999 Silicon Graphics, Inc. All Rights Reserved.
TrademarkInformation